dukDukz
2021.03.31 Javascript 기본기 2_ let과 const 형변환 본문
1. 전역변수와 지역변수
1) 전역변수 선언하고 함수안에서 지역변수로 재정의 했을 때
var index = 10;
function hello(){
var index = 0;
console.log(index);
index++;
}
hello();
console.log(index);
결과 값 : 0
10
함수 안에 var index = 0; 이건 함수 안에서만 작동
console.log(index)는 전역변수를 출력하겠다는 뜻
2) 전역변수 선언하고 재정의하기 전에 콘솔을 찍은 경우
var index = 10;
function hello(){
console.log(index);
var index = 0;
console.log(index);
index++;
}
hello();
console.log(index);
결과 : undefined
0
10
index라는 변수 이름이 같아서 함수 안에서 오류가 남
3) 전역변수의 이름과 지역변수의 이름을 다르게 해서 오류해결
var num = 10;
function hello(){
console.log(num);
var index = 0;
console.log(index);
index++;
}
hello();
console.log(num);
결과 : 10
0
10
전역 변수의 이름을 바꿔주면 원하는 결과 값이 10 0 10을 얻을 수 있음
2. let 과 var
1) for 문 밖에서 i를 호출할때 --> 원래는 안되는거임! -> js는 되고 있음..
for(i=0; i<10; i++){
}
console.log(i);
결과: 10
i=0 앞에 let을 붙여서 선언하면 for문 안에서만 작동된다
그래서 let을 붙이면 결과값이 i가 선언이 안되었다는 오류가 뜬다
2) for문안에 i를 let으로 선언 --> for문 밖에서 호출이 안됨
--> for문 안에서만 호출 가능함
for(let i=0; i<10; i++){
}
console.log(i);
for(let i=0; i<10; i++){
console.log(i);
}
결과 : Error
0 1 2 3 4 .... 9
let num = 10; --> 전역변수로 사용하겠다
function(){
let num = 0; --> 함수 안에서 지역변수로만 사용하겠다
}
그래서 let은 var와 같은 기능을 한다.
+ 전역 변수를 선언할때도 let으로 선언할 수 있다.
let : 변수 선언할 때 사용한다. 사용한 위치에 따라서 사용범위가 달라진다.
3. const
1) const로 전역변수 선언함 --> const는 변하지 않는 값! [index++ 불가]
const index = 0;
function hello(){
console.log(index);
index++;
}
hello();
hello();
결과 :0
uncaught TypeError: Assignment to constant
const는 상수이므로 변하지 않는다. 변하지 않는 값을 넣을 때 (setting값) const를 사용한다.
const는 변하지 않기 때문에 index ++ 를 하면 Error가 뜬다.
(요즘은 var보다 const를 더 많이 사용한다.)
2) const로 전역변수 선언함 --> const는 변하지 않는 값! 2 [for문으로 증가 불가]
const index = 0;
for(index=0; index<10; index++){
console.log(index);
}
결과 : Uncaught TypeError
이유:
const index = 0; 이라고 index값은 변하지 않는 상수라고 선언을 해놓고,
for문으로 index를 0부터 10까지 증가하면서 변하게 한다고 했으니 오류가 난 것
3) 변수선언
const root = document.querySelector('#root');
//root라는 변수는 절대 변하지 않는다.
4. 형변환 해서 div에 찍기
항상 변수 타입에 유의하기
let a = 0; // 변수값안에 ''가 없고 숫자만 있으면 변수타입이 int
let b = '0'; // 변수값 안에 '' 가 있으면 안에 어떠한 값이 들어가든 이 변수 타입은 String
const root = document.querySelector('#root');
const star = "*";
for (let i=0; i<10; i++){
let li = document.createElement('li');
li.innerHTML = String(i+1) + "번 : "+star;
root.appendChild(li);
}
innerHTML 로 뭔가를 넣어주고 싶을 땐, 옆에 String 형태의 글을 써야한다.
그런데 i 라는 변수는 int 형태이므로 int -> string으로 형변환을 해준 뒤 써줘야한다.
형변환 int to string --> String(int변수);
+ root.appendChild(부모 안에 넣을 자식변수)
--> 문법 주의하기
5. 이중 for문
/*별로 10*10 사각형 찍기*/
for (let i=0; i<10; i++){
for(let j=0; j<10; j++){
document.write(star);
}
document.write('<br>');
}
i = 0 일때 j=0~9까지 star를 찍는다.
한번 끝나면 <br>을 찍어서 한줄 띄운다.
i = 9 까지 반복 후 끝남
for (let i=0; i<10; i++){
for(let j=0; j<i; j++){
document.write(star);
}
document.write('<br>');
}
i = 0으로 들어와서 j=0이고 j<i=0 까지 한번 찍어라 --> 안찍히고 br 찍히고 통과됨
그 다음, i = 1 인 상태로 for문에 들어와서 j=0부터 j<i=1까지 한번 찍어라 --> 별 하나 찍고 br 찍히고 통과됨
6. 이중 for문과 배열 비교
1. 별 찍기
// 이중 for문
for (let i=0; i<6; i++){
for(let j=0; j<i; j++){
document.write(star);
}
document.write('<br>');
}
//배열
let arr = ['*', '**', '***', '****', '*****'];
for(let i =0; i<5; i++){
console.log(arr[i]);
}
+ document.write(star+"<br>"); 로 찍은경우
--> for문이 돌때마다 br이 적용되어 한줄에 별 하나만 찍힘
for(i=0; i<=5; i++){
for(j=0; j<=i; j++){
document.write(star+"<br>");
}
}

이렇게 찍으면 j가 하나씩 늘어날때마다 br을 찍기 때문에 별이 한개씩 찍힘
2. 4번째 내용 hello로 변경하기
//이중 for문
for (let i=0; i<6; i++){
for(let j=0; j<i; j++){
if(i!=4){
document.write(star);
}
}
if(i==4){
document.write("hello");
}
document.write('<br>');
}
// 배열
let arr = ['*', '**', '***', 'hello', '*****'];
for(let i =0; i<5; i++){
console.log(arr[i]);
}
+ for 문 안에 if 문을 if else로 쓴 경우 i = 4 인 경우가 n번 반복 되므로 hello가 계속 찍힘
for (let i=0; i<6; i++){
for(let j=0; j<i; j++){
if(i==4){
document.write("hello");
}else{
document.write(star);
}
}
document.write('<br>');
}
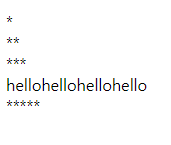
'웹 개발 > JAVASCRIPT' 카테고리의 다른 글
21.04.12 forEach / callback 함수 / 익명함수 (0) | 2021.04.12 |
---|---|
2021.03.31 JS Object 객체란? (0) | 2021.03.31 |
2021.03.30 Javascript 기본 문법 (0) | 2021.03.30 |
2021.03.30 버튼으로 슬라이드 제어 오류_해결 + 슬라이드 정지/재생 (0) | 2021.03.30 |
2021.03.25 경일 배너 만들기 (애니메이션) (0) | 2021.03.25 |